[フロントエンド] axiosライブラリを使って、柔軟にHTTP通信を行う
こんにちは、@yoheiMuneです。
大変久しぶりなブログの更新で少しドキドキです。今日はよくお世話になっているaxiosというHTTPクライアントのライブラリの使い方を、ブログに書きたいと思います。
インストールは簡単で、npm経由で導入できます。
それでは、axiosの機能を具体的に見てみたいと思います。
まずは、以下のようにaxiosを読み込みます。
また、以下のように
https://github.com/yoheiMune/frontend-playground/tree/master/014-axios
https://github.com/mzabriskie/axios
最後になりますが本ブログでは、フロントエンド・Node.js・Linux・インフラ・開発環境・Python・Go言語・Swift・Java・機械学習など雑多に情報発信をしていきます。自分の第2の脳にすべく、情報をブログに貯めています。気になった方は、本ブログのRSSやTwitterをフォローして頂けると幸いです ^ ^。
最後までご覧頂きましてありがとうございました!
大変久しぶりなブログの更新で少しドキドキです。今日はよくお世話になっているaxiosというHTTPクライアントのライブラリの使い方を、ブログに書きたいと思います。
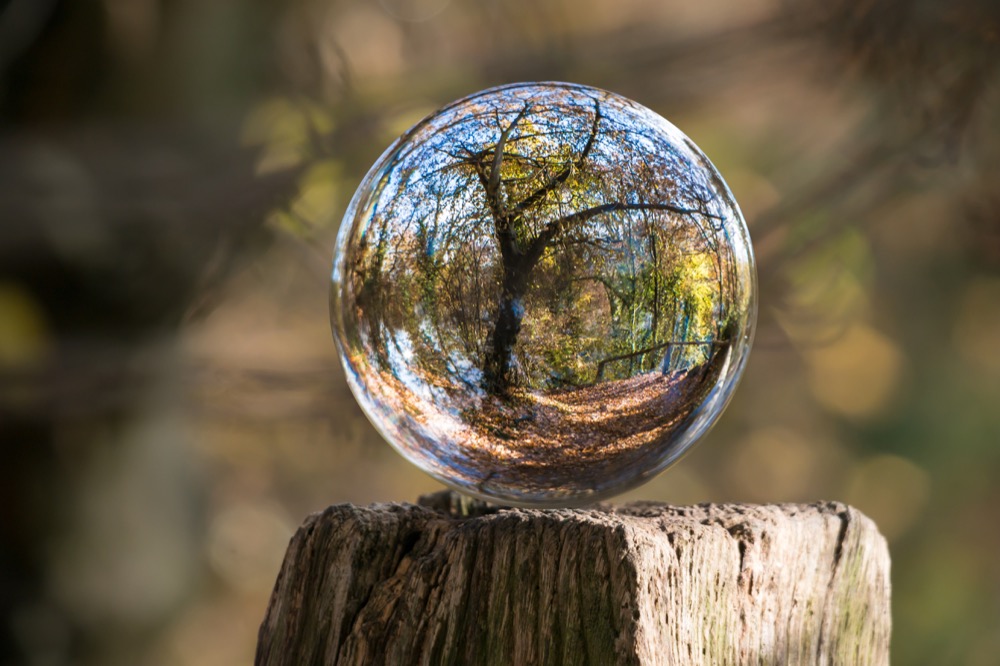
目次
axiosとは
axiosは、HTTP通信を簡単に行うことができるJavascriptライブラリです。本ブログのタイトルには「フロントエンド」と書きましたが、Node.JSでも利用できます。axiosの特徴して以下のような点が挙げられます。axiosの特徴
- XML HttpReqestを簡単に生成できる
- Promiseベースである
- カスタムヘッダーやBasic認証など、いろいろなオプションが手軽にできる
インストールは簡単で、npm経由で導入できます。
$ npm install axios
それでは、axiosの機能を具体的に見てみたいと思います。
axiosを使う
ここからはaxiosの機能のうち、特によく使うものを見てみたいと思います。まずは、以下のようにaxiosを読み込みます。
// requireを使う場合 const axios = require('axios'); // ES6形式の場合 import axios from 'axios';
GET通信を行う
GET通信を行うには、axios.get
関数を用いることで実現できます。// GET通信 axios.get('http://localhost:3000/users') // thenで成功した場合の処理をかける .then(response => { console.log('status:', response.status); // 200 console.log('body:', response.data); // response body. // catchでエラー時の挙動を定義する }).catch(err => { console.log('err:', err); });上記のように、Promiseベースで成功時と失敗時の挙動を定義することができます。
また、以下のように
axios
にconfigオブジェクトを渡す形でも、GET通信を行うことができます。// configオブジェクトを使ったGET通信の例 axios({ method : 'GET', url : 'http://localhost:3000/users' }).then(response => console.log(response.status));
GET通信(パラメータ付き)
GETパラメータはURLにつける(例:/users?name=Yohei
)こともできますが、以下のようにparams
で渡すこともできます。// GET(パラメータ付き) const params = { name : 'Yohei' }; axios.get('http://localhost:3000/users', { params }).then(response => { console.log('status:', response.status); console.log('body:', response.data); }); // configオブジェクト利用の場合 axios({ method : 'GET', url : 'http://localhost:3000/users', params : { name : 'Yohei' } }).then(response => console.log(response.status));
POST通信
POST通信を行う場合には、axios.post
関数を用います。// POST通信 // POSTデータは、axios.postの第2引数で渡します. const data = { firstName : 'Yohei', lastName : 'Munesada' }; axios.post('http://localhost:3000/users', data).then(response => { console.log('body:', response.data); // Yohei Munesada }); // または、configオブジェクトを使う場合 axios({ method : 'POST', url : 'http://localhost:3000/users', data : { firstName : 'Yohei', lastName : 'Munesada' } }).then(response => console.log(response.status));この辺りは、簡単にパラメータも渡せるので便利ですね。
レスポンスの構造
上記でGETやPOSTの扱いを見てきましたが、それらのレスポンスでは以下のような値を取得することができます。// レスポンス構造. axios.get('http://localhost:3000/users').then(function(response) { console.log(response.data); // レスポンスデータ console.log(response.status); // ステータスコード console.log(response.statusText); // ステータステキスト console.log(response.headers); // レスポンスヘッダ console.log(response.config); // コンフィグ });
バイナリーデータのダウンロード
responseType
オプションはデフォルトではjson
ですが、arrayBuffer
を指定することでバイナリーもダウンロードすることができます。// バイナリーデータの取得 axios.get('http://localhost:3000/static/sea001.jpg', { responseType : 'arrayBuffer' }) .then(response => { console.log('response.data', response.data.length); }); // configオブジェクトの場合 axios({ method : 'GET', url : 'http://localhost:3000/static/sea001.jpg', responseType : 'arrayBuffer' }).then(response => { console.log('response.data', response.data.length); });
エラーハンドリング
catch
でエラーを捉えた場合に、以下のようにエラー情報にアクセスすることができます。// エラーハンドリング // ステータスコードが2xx以外だと、エラーとして処理されます. axios.get('http://localhost:3000/error').catch(function (error) { if (error.response) { // The request was made and the server responded with a status code // that falls out of the range of 2xx console.log(error.response.data); console.log(error.response.status); // 例:400 console.log(error.response.statusText); // Bad Request console.log(error.response.headers); } else if (error.request) { // The request was made but no response was received // `error.request` is an instance of XMLHttpRequest in the browser and an instance of // http.ClientRequest in node.js console.log(error.request); } else { // Something happened in setting up the request that triggered an Error console.log('Error', error.message); } console.log(error.config); });エラー状態によって、いくつか取得方法が異なりますが、基本的には
error.response
の中を調べることが多いかなと思います。何をエラーとして扱うかを定義する
デフォルトでは、レスポンスのステータスコードが200系以外だとエラーとして扱われますが、どのステータスコードを正常/異常と扱うかは、独自に定義することができます。axios({ method : 'GET', url : 'http://localhost:3000/error', // 500系以外は正常として扱う(thenで処理できるようにする). validateStatus: function (status) { return status > 500; } }).then(response => console.log(response.status));
タイムアウト
オプションでタイムアウト時間を設定することができます。// タイムアウトを設定 axios.get('http://localhost:3000/users', { timeout : 1000 }) .then(response => console.log(response.status)); // configオブジェクト形式の場合 axios({ method : 'GET', url : 'http://localhost:3000/users', timeout : 1000 // ms }).then(response => console.log(response.status));
カスタムヘッダーの付与
アプリによっては独自のカスタムヘッダをリクエストに付与する必要がありますが、axiosならそれも簡単に行うことができます。// カスタムヘッダー axios.get('http://localhost:3000/users', { headers: {'X-SPECIAL-TOKEN': 'abcde'} }) .then(response => console.log(response.status)); // configオブジェクトの場合 axios({ method : 'GET', url : 'http://localhost:3000/users', headers: {'X-SPECIAL-TOKEN': 'abcde'}, }).then(response => console.log(response.status));
Basic認証
auth
オプションを使うことで、簡単にBasic認証を扱うことができます。// Basic認証 axios.get('http://localhost:3000/users', { auth : { username : 'user1', password : 'pass1' } }) .then(response => console.log(response.status)); axios({ method : 'GET', url : 'http://localhost:3000/users', auth : { username : 'user1', password : 'pass1' }, }).then(response => console.log(response.status));
今回のサンプルコード
今回のクライアントと、サーバー側の実装は以下にコードを置きました。参考になれば幸いです。https://github.com/yoheiMune/frontend-playground/tree/master/014-axios
さらに詳しく
axiosのGithubページにて、オプションなど詳しく説明されていますので、適宜ご確認ください。https://github.com/mzabriskie/axios
最後に
axiosはよく使うのですが、毎度調べてばかりだったので、今回ブログにノウハウをまとめて見ました。すっきりした。これでかなり効率的に使える気がしています。今後も良く使うライブラリはこんな感じでブログに残したいと思います。最後になりますが本ブログでは、フロントエンド・Node.js・Linux・インフラ・開発環境・Python・Go言語・Swift・Java・機械学習など雑多に情報発信をしていきます。自分の第2の脳にすべく、情報をブログに貯めています。気になった方は、本ブログのRSSやTwitterをフォローして頂けると幸いです ^ ^。
最後までご覧頂きましてありがとうございました!